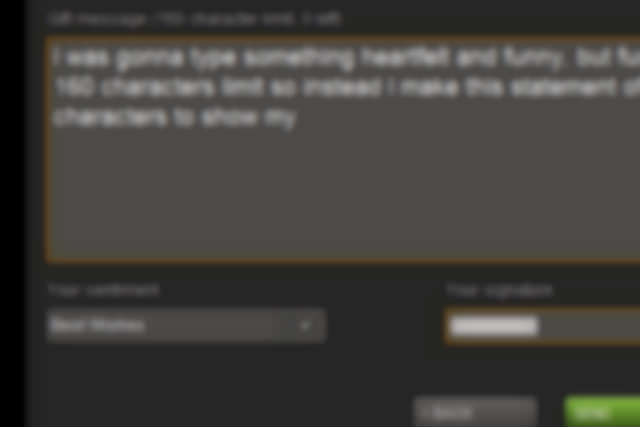
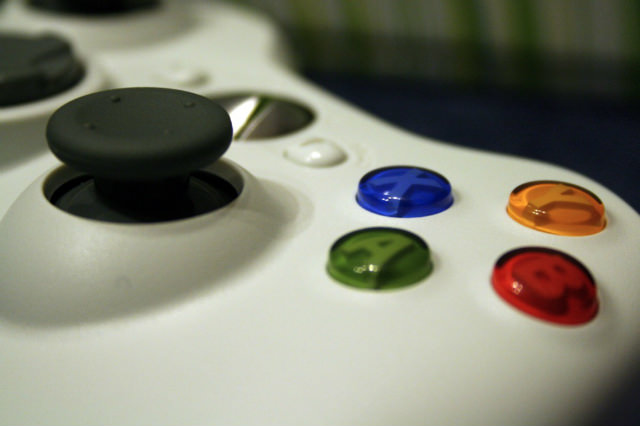
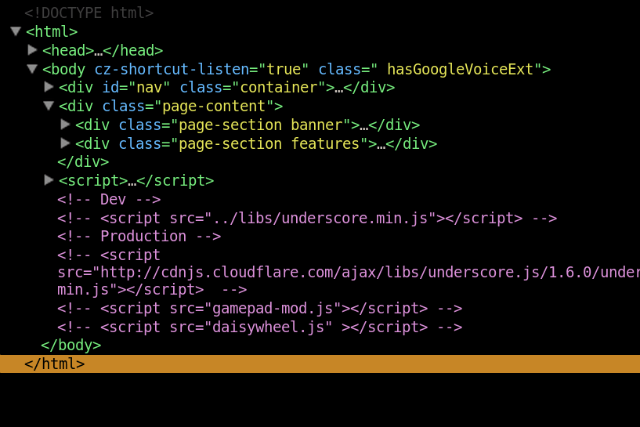
Introduction
DaisywheelJS is a UI tool built for HTML5 games and sites that wish to utilize a 'gamepad' as their users' main input device. It is an alternative to the QWERTY keyboard setup, which is commonly frustrating for those with a controller in hand.
Its radial layout allows the selection of any symbol with just 2 - 3 actions. Compare this to the QWERTY layout that forces the user to navigate to each symbol individually, taking up to 12 actions.
How It Works
This efficiency is achieved by using the left analog stick to choose a group of symbols or a 'petal' and then pressing one of the four colored buttons to select the actual symbol itself.
Controls
- Left Analog Stick: Select group/petal.
- Face Buttons: Select symbol from group/petal.
- Right Shoulder Button: Toggle capital letters.
- Left Shoulder Button: Toggle numbers.
A variety of options allow for custom symbol sets and manually loading the UI. Check out the options and extensions in the docs for implementation.
Docs
Setup
Setup for Daisywheel is simple and easily extendabled. It uses the UMD, meaning it supports install and usage through CommonJS, AMD, and globals. There is an npm package and the script can be found in the dist directory of the Daisywheeljs repo.
CommonJS
var Daisywheel = require('daisywheel');
AMD
define(['daisywheel'], function(Daisywheel) {
...
});
Global
<script src="js/lib/daisywheel.min.js"></script>
Now all that's needed is to include the daisywheel.min.css file, and to add the daisywheel CSS class to whatever input or textarea tag the tool will provide input for.
<link rel="stylesheet" type="text/css" href="css/lib/daisywheel.min.css">
...
<input class="daisywheel">
That's it! Whenever the 'focus' event for that input is fired the Daisywheel UI will automatically appear, allowing the user to enter text with their gamepad without having to switch to their keyboard.
Options and Extensions
Manually loading and unloading
Daisywheel can be manually loaded and unloaded by calling load and unload respectively on the global Daisywheel object. The load function expects a single callback parameter which will return the currently selected symbol.
//When the Daisywheel should appear
Daisywheel.load(function(symbol) {
console.log(symbol);
});
//When the Daisywheel should close
Daisywheel.unload();
Custom symbol sets
A custom set of symbols can be sent into Daisywheel to add support for multiple languages, emojis, etc. The symbols function expects an array of objects, each with a set, an override, and a title attribute.
//Adding Japanese Katakana alphabet
Daisywheel.symbols([{
set: ["あ", "い", "う", "え", "お", "か", "き", "く", "け", ....],
override: true,
title: 'Japanese (Katakana)'
}]);
//Adding Emoji support
Daisywheel.symbols([{
set: [
{
symbol: '🍄'
font: 'EmojiSymbols'
},
{
symbol: '🌞'
font: 'EmojiSymbols'
},
],
override: true,
title: 'Emoji Symbols'
}]);
The set property is the array of symbols (a string or an object) that will be displayed. Simple symbols that only require a single character can be concatenated into a string. If the symbol is not supported by utf-8 (i.e. emojis) then the font attribute within the symbol's object should be set to the font-family of the desired font (remember to include the font file if it is not supported by default).
The override property is a boolean that determines whether the symbol set will 'override' one of the default sets or not. Note: If more than 3 symbol sets have an override property of true the defaults will not be shown at all.
The title property is required if the override property is set. It's the string that's used to label the control for its corresponding symbol set.
Troubleshooting
The Github Wiki is helpful for troubleshooting controller connectivity.
Community
To find out more about DaisywheelJS, or contribute to the project, there are several resources:
Stackoverlfow: General questions and answers, specifics about how-to, etc.
Github: Source code, issue tracking. More details below.
Contributing
Contributions to the library are welcome and encouraged. The source code for the project can be found on Github.
Feature Requests
Discussion is welcomed! If there is a particular feature or API change you'd like to see, create an issue on the project's repo and include any links or images that might be beneficial to the conversation.
About
DaisywheelJS was inspired by the Steam "Big Picture" Daisywheel and the fact that there should never be a QWERTY keyboard presented to a user that has a controller in their hand. This project ports that great solution to Javascript and CSS so the HTML5 gaming community can use it in their games as a standard library/toolkit.
An explanation of how it works can be found here.
Browser Support
As of now, the Gamepad API is only supported by Chrome 21+ and Firefox 29+.
Attributions
gamepad-mod.js interfaces with the browser API and organizes the data sent over in the controller's events and can be found here. It was a modification of the gamepad.js library in the example code for the HTML5Rocks gamepad tester, found in its original form here.
The Montserrat webfont is used for numbers, letters, and all utf-8 characters. It can be found here, accessible through Google webfonts.
License
DaisywheelJS is licensed under the MIT Open-source License.